Javascript
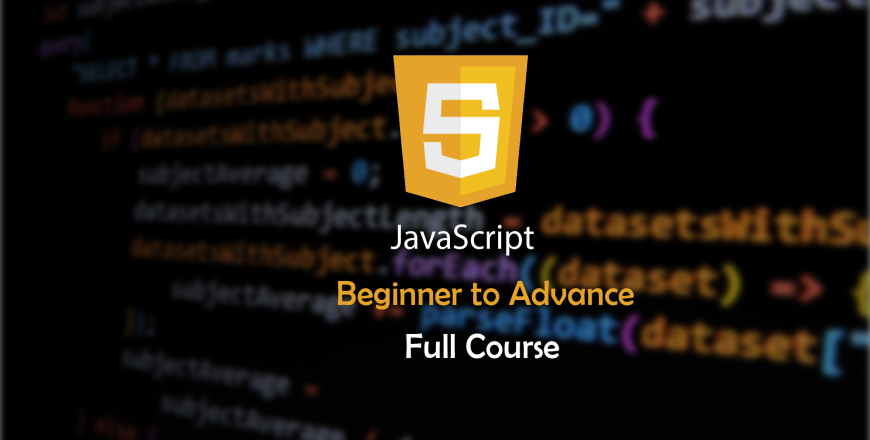
Master the programming language of the web and bring your website to life with our comprehensive Javascript course. Our expert instructors will guide you through the fundamentals of Javascript, from syntax and data types to functions and DOM manipulation, to help you build dynamic and interactive web applications.
COURSE OUTLINE
Getting started
- Goals and objectives
- Course outline
- Computing requirements
- Prerequisites
- How do I communicate?
- How will I learn?
- How will I be graded?
- Navigation buttons
- Menu tree
- Text input exercises
- Browser view exercises
- Browser text input exercises
- Quiz exercises
- How do I start?
The need for scripting
- Scripting overview
- Where do we use scripting?
- Scripting in Web browsers
- JavaScript
- JScript
- ECMAScript
- Java
- What can JavaScript do?
- What can JavaScript not do?
- Browser wars and JavaScript compatibility
- Scripting beyond this course
- JavaScript-based languages
- Web-based languages
Hello world
- Writing Hello World to the screen
- Another method to write to the screen
- Adding JavaScript to an HTML page
- Using JavaScript in the head tag
- Using JavaScript with an HTML element
- Linking to an external script
- Summary
JavaScript basics
- Understanding JavaScript
- Writing JavaScript
- Debugging JavaScript
- Adding comments
- Understanding JavaScript characteristics
- Case-sensitivity
- Reserved words
- Variables
- Variable types
- Quotation marks
- Escaping characters
- JavaScript statements
- JavaScript objects and properties
- JavaScript methods
- JavaScript functions
- JavaScript operators
- Mathematical operators
- Assignment operators
- Comparison operators
- Logical operators
- Bitwise and special operators
- JavaScript expressions
- Review
- Quiz
- Summary
- Getting ready for the exercises
Interacting with the user
- Concepts: variables and conditionals
- JavaScript variables
- Local versus global variables
- If /else statements
- Dialog boxes
- Asking for confirmation
- Prompting
- Using variables to store information
- Adding variables to strings
- Alerts
- Debugging with alerts
- Quiz
- Summary
JavaScript events
- Calling JavaScript from HTML: Events
- Concepts: parameters and thethis keyword
- Using parameters in a function
- The thiskeyword
- Page load events
- Event: onload
- Event: onunload
- Form events
- Events: onfocus, onblur
- Event: onchange
- Events: onsubmit, onreset
- Mouse events
- Event: onclick
- Events: onmouseover, onmouseout
- Events: onmousedown, onmouseup
- Combining events and CSS properties
- Common events and event handlers
- Quiz
- Summary
Working with forms
- Quick review of forms
- Concepts: return, parseFloat, Arrays, and isNaN
- Return command
- Using parseFloat and parseInt
- isNaN function
- Arrays
- Referring to forms in JavaScript
- Changing the value of a field in a form
- Creating drop-down links
- Using checkboxes in forms
- Using radio buttons
- Reading values entered in a form
- Doing form calculations
- Form input validation
- Why validate with JavaScript?
- Checking user input in a form
- Using the return command in validation
- Form validation – checking for a minimum number
- Checking for an empty field using onblur
- Validating an email address
- Quiz
- Summary
Working with objects
- Object hierarchy
- Assigning properties to an object
- Using methods to manipulate objects
- Creating a new custom object
- Concepts: eval and length
- Length property
- evalfunction
- Window object
- Opening a pop-up window
- Enlarging an image
- Creating a simple timer
- Document object
- String object
- Joining strings – concatenation
- Fetching characters
- Finding and fetching pieces
- Splitting a string
- Arrays
- Setting and getting values
- Joining arrays
- Date object
- Today
- Getters and setters
- Message with today’s date
- Local time, UTC
- Math object
- Image object
- Image slideshow
- Summary
Changing HTML with JavaScript
- Concepts: conditions and loops
- If/else statement
- While loop
- For loops
- Do/while loop
- Changing properties
- Changing image properties
- Writing HTML dynamically
- Doing mathematical calculations
- Doing conversions
- Creating a simple order form
- Creating new Web pages using JavaScript
- Using rollovers
- Putting events and properties together: image rollovers
- Using image rollovers with links
- More scenarios
Using the DOM
- More about the DOM tree
- Understanding the DOM
- Accessing the DOM tree
- Using the DOM in Web pages
- Using styles with DOM
- Finding elements
- Changing attributes
- Creating elements
- Setting a time delay
- Hiding and displaying text
- Cookies
- Cookie property
- Remembering values between events
- Remembering values with a global variable
- Remembering values with a DOM property
- Quiz
- Summary
JavaScript “recipes”
- Identifying browsers
- Using links to execute JavaScript
- Opening a popup in the center of the page
- Viewing a directory
- Detecting screen resolution
- Checking and unchecking checkboxes
- Window status bar
- Counting the characters in a form field
Be the first to add a review.
Please, login to leave a review